Getting Started With V
Hit the ground running with this collection of resources
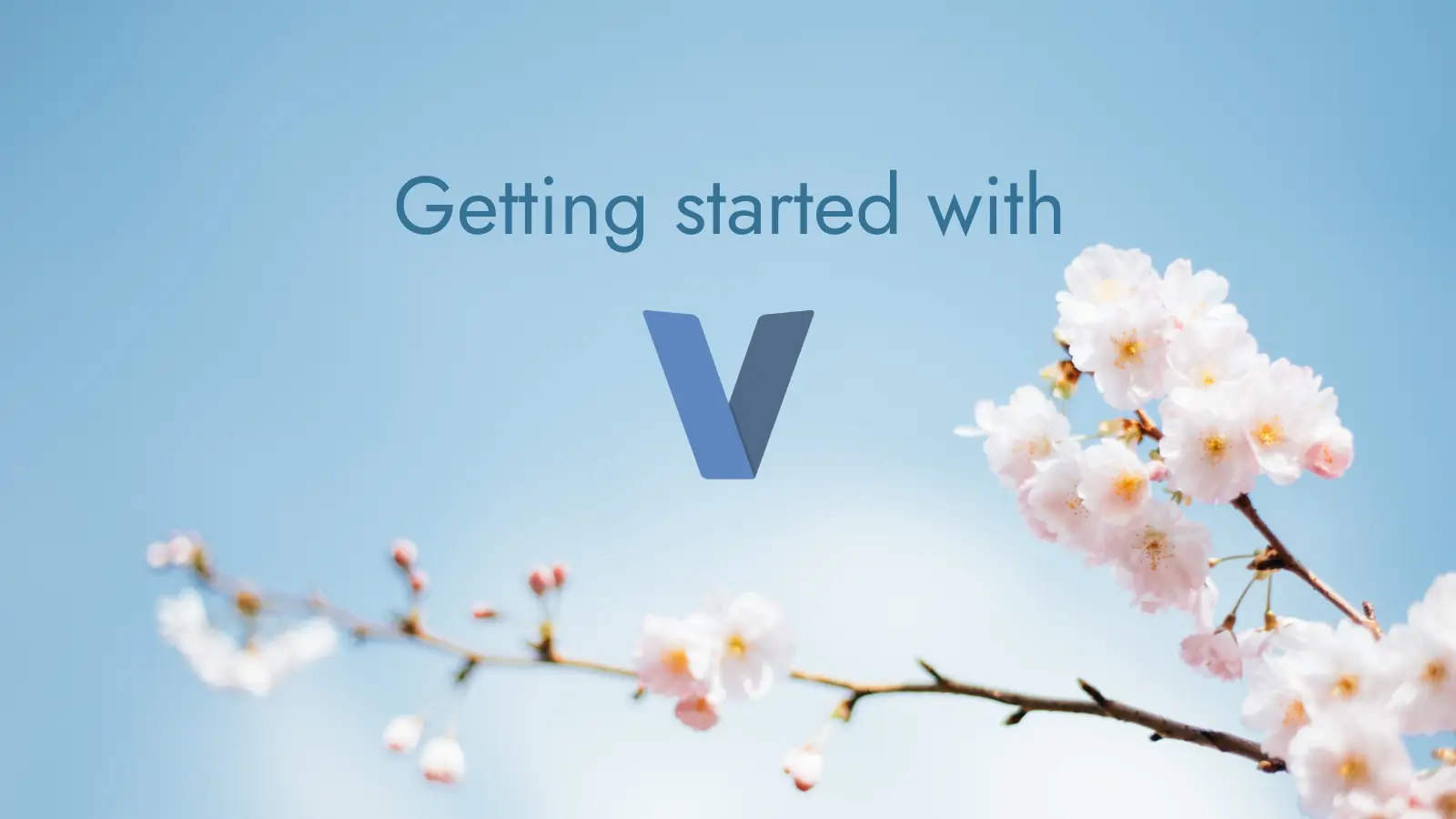
Introduction
V is a simple, fast, safe, compiled general purpose programming language for developing maintainable software. There are several compelling reasons for using V, which you can learn more about here: The V Programming Language
In this post, we focus on getting new users up to speed so that they can start working with V right away.
Installing (and Updating) V
Since V is in active development, there will be a lot of breaking changes often. Therefore, it is recommended that you install V from source.
Installing V from source
git clone https://github.com/vlang/v
cd v
make # make.bat on Windows
V compiles itself really fast and requires no dependencies and libraries for self compilation. For more information, see Installing V from source. You can optionally leverage established C compilers like GCC, Clang, or MSVC to generate optimized binaries.
Weekly Release
V makes weekly releases (besides the normal semantic version update releases) if you do not prefer compiling from source. You can find the releases here: Releases.
Symlinking
The V executable generated (or obtained) is not automatically added to your PATH. V provides a convenient solution to this.
# On Linux, Mac OS, and other similar systems
sudo ./v symlink
# On Windows, open an Administrator shell and run the following.
# NOTE: This is the ONLY command that neeeds to be run from an Administrator shell.
.\v.exe symlink
Refer to this section for more information: Symlinking.
Updating V
Similar to rustup
, V supports the following command:
v up
This command updates the V compiler to the latest version available from the main Github repository.
Learning V
Syntactically, V is similar to Go. Therefore GoLang devs can feel at home when they write V code. Knowledge of Go is not necessary; the language is very simple and the main concepts can be learned over a weekend.
The official documentation for the language is written in a single markdown document: V Documentation.
The documentation for all of the modules included in the V standard library (vlib) is available here: vlib Documentation. You are encouraged to use the search bar to find the functions and structs you need for your project.
For a taste of V here is a truncated example of TOML file parsing. The sample TOML file is available here: TOML Example
|
|
Source code: toml.v
Setting up your Development Environment
Code Editor
You are free to use any code editor you prefer. We recommend Visual Studio Code for beginners because we support it officially through the VSCode extension. For help with other editors, please join our Discord Server.
Language Server
The V Language Server (WIP) is an LSP compatible language server. The VSCode extension should be able to automatically download and install VLS. In case of errors, check out the pre-built binaries, or build VLS from source. If your find any bugs, get them confirmed in the #vls channel on our server and then report them on Github. We are also open to contributions!
Running a V Program
Your project should have at least one main
function. Then you can compile and run
you code in one of two ways:
- Directly run the program with
v run file.v
orv run directory
. You can even dov run .
to run the only main function in the directory. - Compile the program with
v file.v
(optionally asv -prod file.v
) and then run the generated executable.
Miscellaneous
You need git
to clone and update V. Make sure that it is installed on your machine.
Additionally, use v new
and v init
as necessary, and use v fmt -w /path/
to automatically format your source code. Consult v help init
and v help fmt
for more information.
Getting Help and Reaching Out
It is recommended that you make frequent use of v help
, consult the
language documentation
and the
modules documentation.
If you want to get specific help, reach out to us directly, or just hang out with
the V community members, join our Discord Server. Use the
appropriate channels for your needs.
While developing in V, you may encounter bugs. Please report them in the #bugs channel, and file Github issues about them with v bug file.v
.